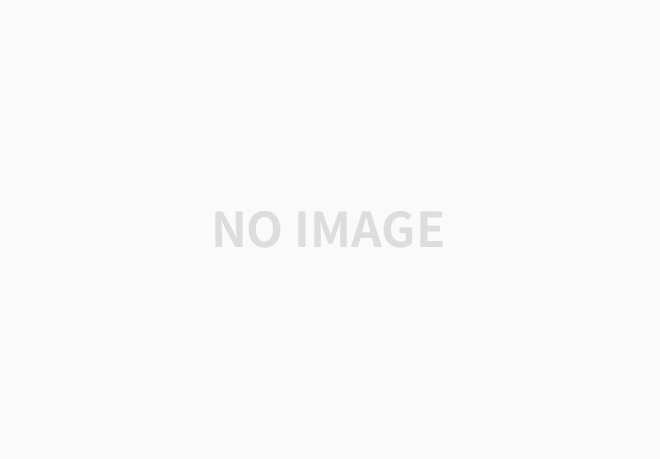
안녕하세요
이번 시간에는 Retrofit 통신에 대해 알아보겠습니다.
Retrofit은 http 통신을 쉽게 사용할 수 있게 만든 라이브러리입니다.
현업에서 많이 사용한다고 하니 기본적인 것은 꼭 알아두는 것이 좋을 것 같습니다.
여기에 있는 JSON형태의 데이터들을 가져올 것입니다.
https://jsonplaceholder.typicode.com/posts/1
우선 Gradle에 다음과 같은 내용을 추가해 줍니다.
//1
implementation 'com.squareup.retrofit2:retrofit:2.6.4'
//2
implementation 'com.squareup.retrofit2:converter-gson:2.6.4'
1. Retrofit을 사용하겠다는 의미입니다.
2. JSON 데이터들을 자바 오브젝트로 변환해 주는 라이브러리입니다.
서버에서 데이터를 받아오면 그것을 자바에서 사용할 수 있게 변환해주는 것입니다.
<uses-permission android:name="android.permission.INTERNET"/>
인터넷 권한을 설정해 줍니다.
public class PResult {
@SerializedName("userId")
private int userId;
@SerializedName("id")
private int id;
private String title;
@SerializedName("body")
private String bodyValue;
@Override
public String toString(){
return "결과{"+
"userId = " + userId + "\n" +
"id = " + id + "\n" +
"title = " + title + "\n" +
"bodyValue = " + bodyValue +
"}";
}
}
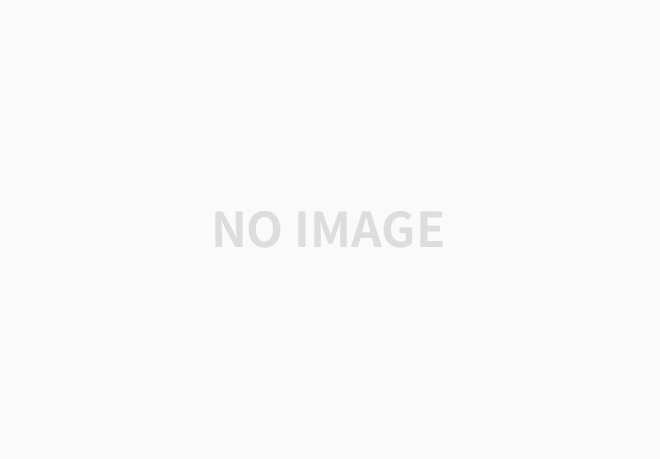
우리가 가져올 JSON 데이터는 이렇게 생겼습니다.
@SerializedName("userId")
private int userId;
@SerializedName를 사용해 userId(첫 번째 줄)를 userId(두 번째 줄)라는 이름으로 사용하겠다는 의미입니다.
만약 @SerializedName("userId123") 이런 식으로 작성하면 우리가 가져올 JSON 데이터의 userId는 null값으로 가져올 것입니다.
@SerializedName를 사용하려면 JSON 데이터의 이름을 정확히 입력해주어야 합니다.
private String title;
JSON 데이터의 이름을 정확히 입력 해주면 @SerializedName를 사용하지 않아도 정상적으로 작동합니다.
public interface RetrofitService {
@GET("posts/{post}")
Call<PResult> getPosts(@Path("post") String post);
}
Http 메소드 중에 Get을 사용하겠다는 의미입니다.
@GET("posts/{post}") 여기에서 posts는 baseUrl을 제외한 EndPoint(URI) 부분을 의미합니다.
우리가 데이터를 가져올 주소를 보면 다음과 같습니다.
https://jsonplaceholder.typicode.com/posts/1
https://json...code.com 까지가 baseUrl(유알엘)이고 나머지 부분을 EndPoint(URI(유알아이))라고 합니다.
Call<Result> getPosts(@Path("post") String post)에서
@Path의 값은 @GET에 중괄호와 같은 이름으로 작성해줍니다.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://jsonplaceholder.typicode.com/") // baseUrl 입력
.addConverterFactory(GsonConverterFactory.create()) // JSON데이터를 Gson으로 변환
.build();
RetrofitService service = retrofit.create(RetrofitService.class);
Call<PResult> call = service.getPosts("1"); //사용할 메소드 선언
call.enqueue(new Callback<PResult>() { // enquene로 비동기 통신, 통신이 완료된 후
// 이벤트 처리를 위한 Callback 리스너 등록
@Override
public void onResponse(Call<PResult> call, Response<PResult> response) {
if(response.isSuccessful()){ //통신 성공 시 처리되는 부분
PResult result = response.body();
Log.e("test","성공 \n" + result.toString());
}
}
@Override
public void onFailure(Call<PResult> call, Throwable t) {
Log.e("test","fail "+t.getMessage()); //통신 실패 시 처리되는 부분
}
});
}
주석으로 작성된 설명을 참고해주세요
다음과 같은 결과를 확인할 수 있습니다.
결과{userId = 1
id = 1
title = sunt aut facere repellat provident occaecati excepturi optio reprehenderit
bodyValue = quia et suscipit
suscipit recusandae consequuntur expedita et cum
reprehenderit molestiae ut ut quas totam
nostrum rerum est autem sunt rem eveniet architecto}
그러면 여러분들이 위에 내용을 조금 변경해서 아래 주소에서 데이터를 가져오는 것을 만들어 보세요
주소
https://jsonplaceholder.typicode.com/todos/1
결과는 다음과 같이 나와야 합니다.
2022-11-19 16:48:09.321 30126-30126/com.psw.retro2 E/test: 성공
결과{userId = 1
id = 1
title = delectus aut autem
completed = false}
정답 ↓
@SerializedName("userId")
private int userId;
@SerializedName("id")
private int id;
private String title;
@SerializedName("completed")
private String completed;
@Override
public String toString(){
return "결과{"+
"userId = " + userId + "\n" +
"id = " + id + "\n" +
"title = " + title + "\n" +
"completed = " + completed +
"}";
}
@GET("todos/{td}")
Call<PResult> getPosts(@Path("td") String td);
MainActivity는 baseUrl이 같아서 변경되는 것이 없음...
수고하셨습니다!
'안드로이드' 카테고리의 다른 글
안드로이드 Retrofit 통신#2 리사이클러뷰 (0) | 2022.11.20 |
---|---|
안드로이드 RxJava #8 RxJava 연산자-(결합) (0) | 2022.11.10 |
안드로이드 RxJava #7 RxJava 연산자-(필터링) (0) | 2022.11.09 |
안드로이드 RxJava #6 RxJava 연산자-(변형) (0) | 2022.11.08 |
안드로이드 RxJava #5 마블 다이어그램, RxJava 연산자-(생성) (0) | 2022.11.07 |