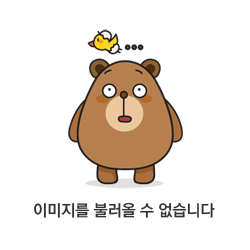
안녕하세요
이번 시간에는 이전에 Retrofit 통신한 것을 조금 변경해서 가져온 데이터들을 리사이클러뷰에 출력해보겠습니다.
https://adc6981.tistory.com/23
안드로이드 Retrofit 통신 사용방법
안녕하세요 이번 시간에는 Retrofit 통신에 대해 알아보겠습니다. Retrofit은 http 통신을 쉽게 사용할 수 있게 만든 라이브러리입니다. 현업에서 많이 사용한다고 하니 기본적인 것은 꼭 알아두는 것
adc6981.tistory.com
Retrofit통신과 리사이클러뷰 사용방법은 이전 블로그에 설명이 되어 있으니 오늘은 코드만 업로드하겠습니다.
https://jsonplaceholder.typicode.com/todos
여기서 JSON 데이터를 가져올 겁니다.
주석에 설명을 해놨으니 참고해 주세요
화면 구성
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".View.MainActivity" >
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
리사이클러뷰에 들어갈 화면 구성
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:orientation="vertical">
<TextView
android:id="@+id/userId"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:text="유저아이디"
android:textSize="20sp" />
<TextView
android:id="@+id/id"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:text="아이디"
android:textSize="14sp" />
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:text="제목"
android:textSize="14sp"
/>
<TextView
android:id="@+id/completed"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:text="트루뽈스"
android:textSize="14sp"
/>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="#000000" />
</LinearLayout>
MainActivity
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recyclerView = findViewById(R.id.recyclerView);
linearLayoutManager = new LinearLayoutManager(this);
recyclerView.setLayoutManager(linearLayoutManager);
arrayList = new ArrayList<>();
recyclerViewAdapter = new RecyclerViewAdapter(arrayList,getApplicationContext());
recyclerView.setAdapter(recyclerViewAdapter);
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://jsonplaceholder.typicode.com/")
.addConverterFactory(GsonConverterFactory.create())
.build();
RetrofitService service = retrofit.create(RetrofitService.class);
for (int i = 1; i < 10; i++) {
String str_i = String.valueOf(i);
Call<PostResult> call = service.getPosts(str_i);
call.enqueue(new Callback<PostResult>() {
@Override
public void onResponse(Call<PostResult> call, Response<PostResult> response) {
if(response.isSuccessful()){
PostResult postResult = new PostResult();
PostResult result = response.body();
postResult.setUserId(result.getUserId());
postResult.setId(result.getId());
postResult.setTitle(result.getTitle());
postResult.setCompleted(result.getCompleted());
arrayList.add(postResult);
Collections.sort(arrayList,new SortByDate()); //arrayList를 정렬
recyclerViewAdapter.notifyDataSetChanged(); //리사이클러뷰 새로고침
}
else{
Log.e("test","실패");
}
}
@Override
public void onFailure(Call<PostResult> call, Throwable t) {
Log.e("test","fail "+t.getMessage());
}
});
}
}
class SortByDate implements Comparator<PostResult> {
@Override
public int compare(PostResult postResult, PostResult t1) {
return postResult.getId().compareTo(t1.getId());
}
}
Adapter
public class RecyclerViewAdapter extends RecyclerView.Adapter<RecyclerViewAdapter.CustomHolder> {
private ArrayList<PostResult> arrayList;
public Context context;
public RecyclerViewAdapter(ArrayList<PostResult> arrayList, Context context) {
this.arrayList = arrayList;
this.context = context;
notifyDataSetChanged();
}
@NonNull
@Override
public RecyclerViewAdapter.CustomHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item,parent,false);
CustomHolder customHolder = new CustomHolder(view);
return customHolder;
}
@Override
public void onBindViewHolder(@NonNull RecyclerViewAdapter.CustomHolder holder, int position) {
String str_userId = String.valueOf(arrayList.get(position).getUserId());
holder.userId.setText(str_userId);
holder.id.setText(arrayList.get(position).getId());
holder.title.setText(arrayList.get(position).getTitle());
holder.completed.setText(arrayList.get(position).getCompleted());
holder.itemView.setTag(position);
holder.itemView.setOnClickListener(new View.OnClickListener() { //클릭시 현재 id값을 출력
@Override
public void onClick(View view) {
String current_id = holder.id.getText().toString();
Toast.makeText(view.getContext(), current_id, Toast.LENGTH_SHORT).show();
}
});
}
@Override
public int getItemCount() {
return arrayList.size();
}
public class CustomHolder extends RecyclerView.ViewHolder {
protected TextView userId;
protected TextView id;
protected TextView title;
protected TextView completed;
public CustomHolder(@NonNull View itemView) {
super(itemView);
this.userId = itemView.findViewById(R.id.userId);
this.id = itemView.findViewById(R.id.id);
this.title = itemView.findViewById(R.id.title);
this.completed = itemView.findViewById(R.id.completed);
}
}
}
MVVM패턴 기준 통신하는 부분은 Model에 있어야 하며 MainActivity에서는 화면 업데이트만 해주는 것이 좋습니다.
다음 시간에는 지금 까지 했던 내용을 참고해서 MVVM패턴을 적용해 Retrofit통신을 해보겠습니다.
수고하셨습니다!
반응형
'안드로이드' 카테고리의 다른 글
안드로이드 Retrofit 통신 사용방법 (0) | 2022.11.19 |
---|---|
안드로이드 RxJava #8 RxJava 연산자-(결합) (0) | 2022.11.10 |
안드로이드 RxJava #7 RxJava 연산자-(필터링) (0) | 2022.11.09 |
안드로이드 RxJava #6 RxJava 연산자-(변형) (0) | 2022.11.08 |
안드로이드 RxJava #5 마블 다이어그램, RxJava 연산자-(생성) (0) | 2022.11.07 |